The Never and NoReturn Annotations in Python
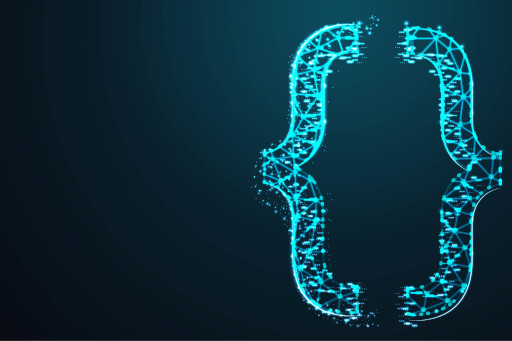
Diving Deeper into the Never and NoReturn Annotations in Python: Enhancing Code Quality and Error Handling
Type annotations in Python are a powerful tool that not only helps developers understand each other's intentions but also provides the opportunity for static code checking and quality improvement. Among the many annotations, Python offers two particularly interesting ones: Never
and NoReturn
. What do they represent and how can their use aid in development?
The NoReturn
Annotation
The introduction of the NoReturn
annotation in Python 3.5 opened up the possibility for developers to explicitly indicate that a function would never return control in the normal way, i.e., it would either raise an exception or terminate the program execution. This is particularly useful for functions like sys.exit()
or os._exit()
, where the return type is irrelevant because there is no return at all.
1from typing import NoReturn
2
3
4def abort() -> NoReturn:
5 raise Exception("Critical Error")
Using NoReturn
improves code readability and aids static analysis tools like Mypy in more effectively detecting errors.
The Never
Annotation in Python 3.11
With the release of Python 3.11, a new annotation Never
was introduced, which serves as a refinement of NoReturn
. It is used to denote functions that not only do not return control (similar to NoReturn
) but also do not take any arguments. This adds an extra layer of clarity when defining API interfaces where the behavior of the function should be absolutely unambiguous.
1def main() -> Never:
2 ...
Applying Annotations to Improve Error Handling
The Never
and NoReturn
annotations play a key role in error handling. They allow developers to clearly document their intentions regarding how functions should behave in exceptional circumstances. This, in turn, helps prevent unforeseen behavior in the code and simplifies debugging.
For example, the NoReturn
annotation can be used for wrapper functions that handle all possible exceptions and should not return control to the calling code:
1def error_handler() -> NoReturn:
2 try:
3 # attempt to perform an operation
4 except Exception as e:
5 log.error(e)
6 sys.exit(1)
Conclusion
The Never
and NoReturn
annotations in Python are powerful tools for enhancing code quality and error handling. They not only improve code readability but also contribute to more effective use of static analysis tools. Utilizing these annotations helps developers clearly express their intentions and ensure more reliable program behavior.